
Why is Persistence Layer Important? (+ a Quick NHibernate Sample)
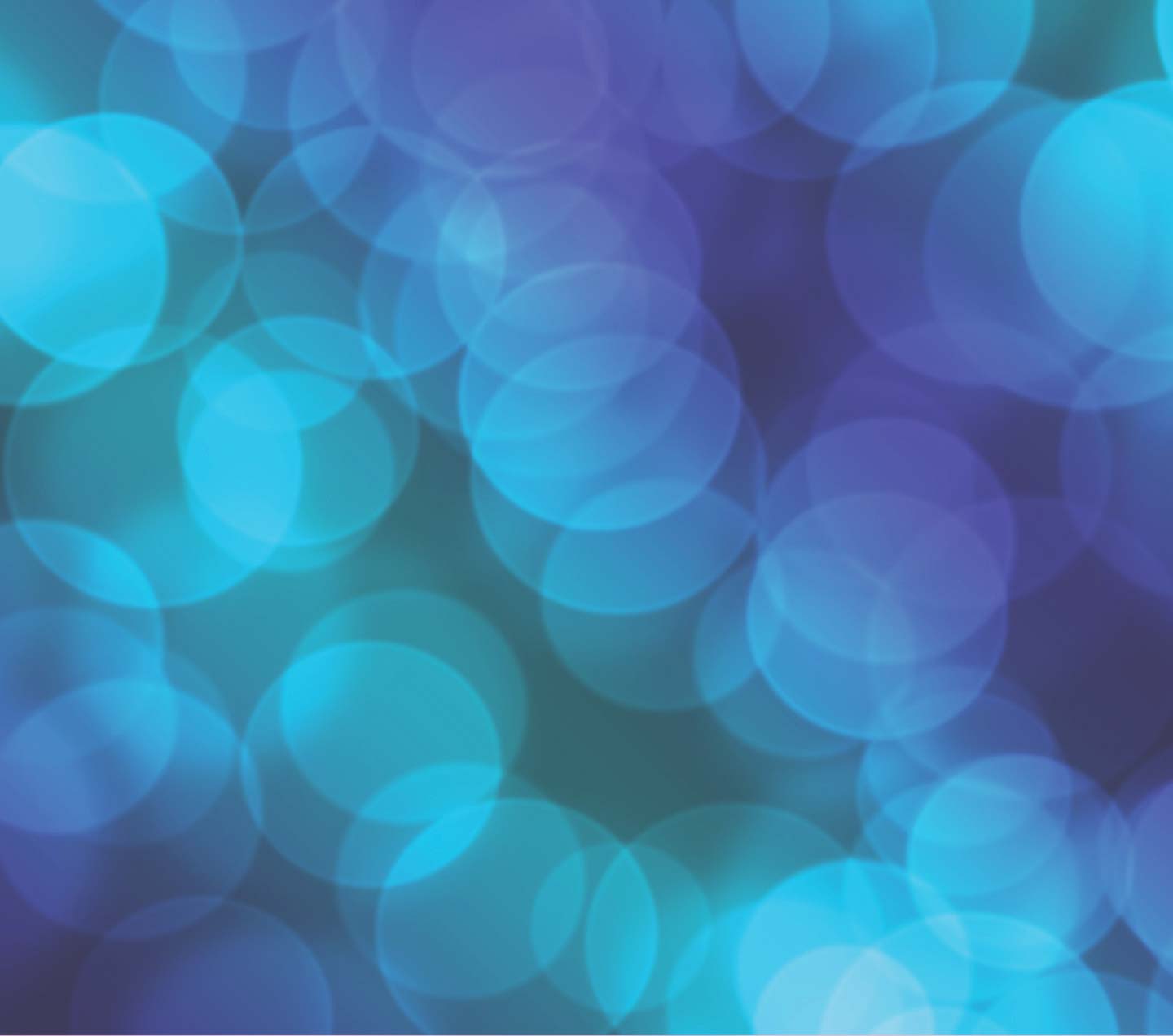

Nov 09, 2011
Let’s investigate the importance of the use of persistence layers between applications and databases. The idea is to show the benefits and functions of persistence layers with a simple example built in NHibernet for .NET applications.
The idea of the persistence layer is to encapsulate databases access routines. This allows applications to work with a set of objects (Data Objects) that read and save their state to a database; therefore applications do not need to have it in their source code SQL statements. The big advantage of persistence layers is to avoid the direct communication between applications and relational databases. A big problem can exist if you do not have a persistence layer because if the database engine is updated or replaced, changes to the http://www.redhat.com/docs/manuals/rhea/RHEA-5.0-Manual/developer-guide/ch-persistence.html
Persistence and Data Objectsapplication source code are required to make it work with the updated database engine.
Following is an example of how to use NHibernate to achieve that separation between applications and relational databases. NHibernate is .NET persistence library for relational databases that can be used in web and console applications to access relational databases. The following example is build for a web application in Visual Studio 2008 with SQL Server 2005:
Project Setup
Please download the last distribution of NHibernate.
Open your Visual Studio and create a .NET Web Application project in C# and named it as "NHibernateSample".
For simplicity, let's create a Persistence project adding to the recently created project that is going to contain the model and the persistence.
In the NHibernateSample project, add a reference to the Persistence project.
The Persistence project is going to use Nhibernate, for that reason, add following references:
NHibernate.dll
,NHibernate.ByteCode.LinFu.dll
andLinFu.DynamicProxy.dll
.
Database Setup
For this part, I am assuming that you already have SQL Server 2005 installed.
Open SQL Server Management Studio and create new database named as "NHibernateDatabase".
Run the following query to create the table users:
use NHibernateDatabase go CREATE TABLE users ( LogonID nvarchar(20) NOT NULL default '0', Name nvarchar(40) default NULL, Password nvarchar(20) default NULL, EmailAddress nvarchar(40) default NULL, LastLogon datetime default NULL, PRIMARY KEY (LogonID) ) go
NHibernate Configuration
Now is time to do the lasts configurations in order to use NHibernate.
Create a .NET class in the project Persistence that is going to represent the table Users in .NET.
using System; namespace Persistence { /// /// Summary description for User /// public class User { private string id; private string userName; private string password; private string emailAddress; private DateTime lastLogon; public User() { } public virtual string Id { get { return id; } set { id = value; } } public virtual string UserName { get { return userName; } set { userName = value; } } public virtual string Password { get { return password; } set { password = value; } } public virtual string EmailAddress { get { return emailAddress; } set { emailAddress = value; } } public virtual DateTime LastLogon { get { return lastLogon; } set { lastLogon = value; } } } }
Add to the Persistence Project the following mapping file named Users.hbm.xml:
<?xml version="1.0" encoding="utf-8" ?> <hibernate-mapping xmlns="urn:nhibernate-mapping-2.2" > <class name="Persistence.User, Persistence" table="users"> <id name="Id" column="LogonId" type="String" length="20"> <generator class="assigned" /> </id> <property name="UserName" column="Name" type="String" length="40"/> <property name="Password" type="String" length="20"/> <property name="EmailAddress" type="String" length="40"/> <property name="LastLogon" type="DateTime"/> </class> </hibernate-mapping>
Right click over the file "User.hbm.xml", properties, and change the Build Action to Embedded Resource.
Open the web.config file of the web project and add the following:
<?xml version="1.0"?> <configuration> <configSections> <section name="hibernate-configuration" type="NHibernate.Cfg.ConfigurationSectionHandler, NHibernate"/> </configSections> <hibernate-configuration xmlns="urn:nhibernate-configuration-2.2"> <session-factory> <property name="connection.provider">NHibernate.Connection.DriverConnectionProvider</property> <property name="connection.isolation">ReadUncommitted</property> <property name="show_sql">true</property> <!-- SQLServer --> <property name="dialect">NHibernate.Dialect.MsSql2005Dialect</property> <property name="connection.driver_class">NHibernate.Driver.SqlClientDriver</property> <property name="connection.connection_string">Server=localhost;initial catalog=nhibernatedatabase;Integrated Security=SSPI</property> <property name="default_schema">NHibernateDatabase.dbo</property> <property name="proxyfactory.factory_class">NHibernate.ByteCode.LinFu.ProxyFactoryFactory, NHibernate.ByteCode.LinFu</property> <mapping assembly="Persistence"/> <!-- MySQL --> </session-factory> </hibernate-configuration> </configuration>
NHibernate Use
Create class called "TestNHibernate.cs" in the Persistence Project to use NHibernate.
solr.in.cmd Uncomment
using System; using System.Collections.Generic; using System.Linq; using System.Text; using NHibernate; using NHibernate.Cfg; namespace Persistence { public class ConfigurationObject { public ConfigurationObject() { Configuration cfg = new Configuration(); cfg.AddAssembly("Persistence"); ISessionFactory factory = cfg.BuildSessionFactory(); ISession session = factory.OpenSession(); ITransaction transaction = session.BeginTransaction(); //Use User newUser = new User(); newUser.Id = "test"; newUser.UserName = "test"; newUser.Password = "abc123"; newUser.EmailAddress = "test@cool.com"; newUser.LastLogon = DateTime.Now; // Save session.Save(newUser); // Comit transaction.Commit(); session.Close(); } } }
Create a class in the NHibernatSample project to call the configuration object.
solr.in.cmd Uncomment
TestNHibernate co = new TestNHibernate ();
The interesting part of persistence is that if we change the database, we just need to change the configuration file located in web.config in order to point to the updated database. Notice that in the code we do not have any SQL statement,because NHibernate is working as the persistence layer that transform calls to the object update to SQL Statements.
Recent Insights
-
-
-
-
Leonardo Bravo
Monitoring and Logging in DevOps
Ensuring Application Performance and Reliability