
.Net Distributed Caching Solution
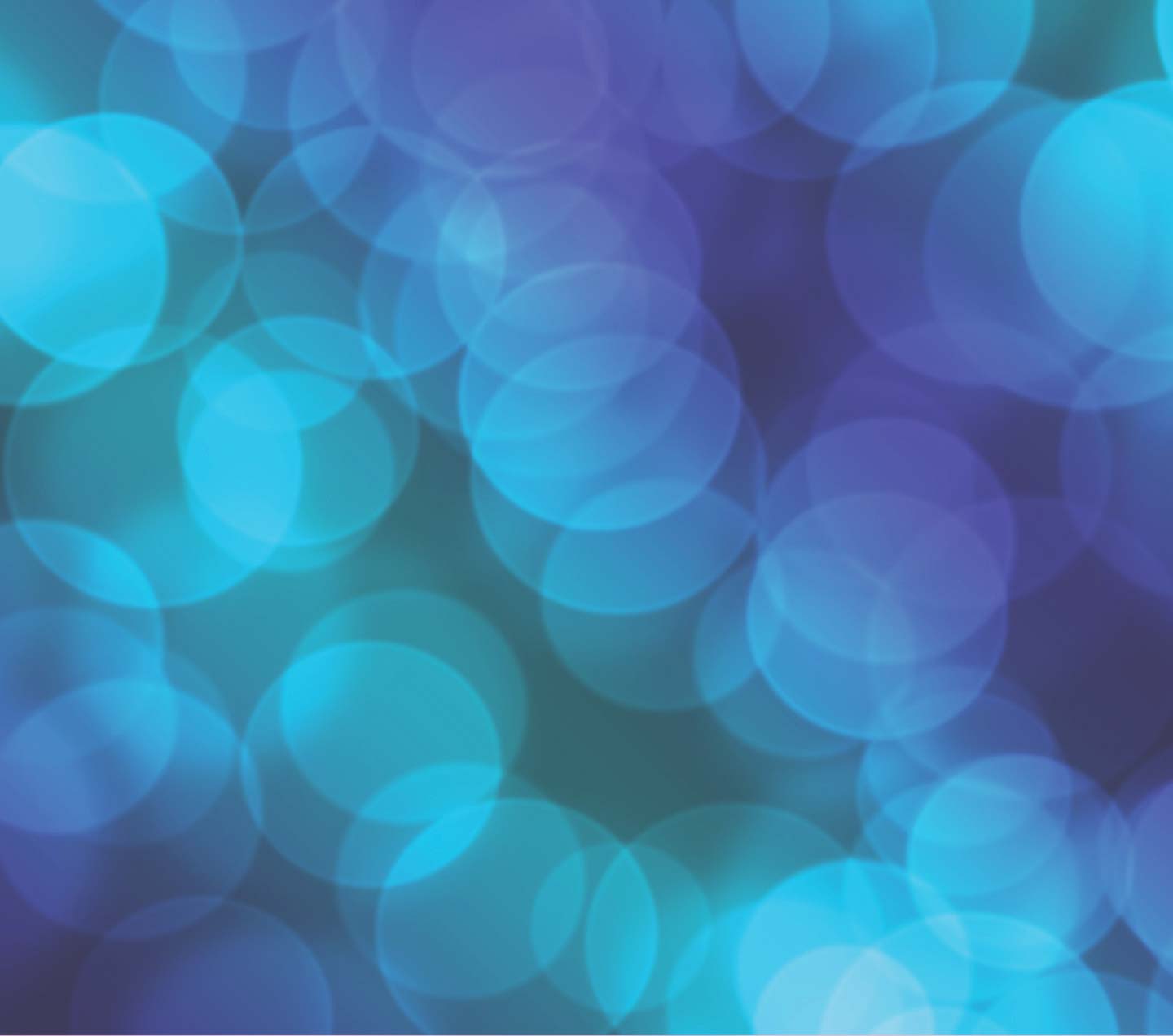

Jun 25, 2012
Installation of Windows Server AppFabric Distributed Caching Solution
Before we can go into the details of these, lets look at typical installation process. Typical installation would involve the following
-
There is a pre-requisite package for installation of Windows Server AppFabric. It requires Web Deploy package that can be downloaded and installed from Microsoft.
-
Requires .NET Framework 4.0 for Server component, but the client component can be installed on .NET Framework 3.5 SP1
-
AppFabric Cache Server (includes the client) Download
-
Install the software packages with admin privileges. Here is a screen showing the installation options you need to select while installing the caching services
-
-
On the Server, you would selct the Caching Services, and Caching Administration. On the Client, you generally install the Cache Client option. For our example since my client and server are the same, I have all the three options selected.
This would install the AppFabric software on your machine. The next step in the process is to create a cache cluster and add hosts to it.
Configuration Options for Windows Server AppFabric Distributed Caching Solution
In this section, we will discuss the various configuration options for AppFabric Distributed Caching Solution such as XML Storage Provider, and SQL Storage Provider and the differences between the two.
In this section, we would be talking about the Configuration Options for AppFabric. AppFabric Configuration Involves the following components. Once the installation is complete, go to Start -> All programs -> Windows Server AppFabric -> Configura AppFabric option.
There are two options of where you can store your Cluster Configuration Store Provider:
XML VS SQL Server Configuration Store Provider. These options support specific settings of cluster configuration. Below is mentioned are the available options with each.
XML Storage Provider | SQL Server Storage Provider | |
---|---|---|
Storage Provider | XML | SQL Server Configuration database |
Configuration Storage Location | UNC share, ex:\\OSHYN\AppFabricShare | SQL Server Configuration Database that gets created during configuration |
Caching Service Account | Local Machine user account, ex: OSHYN\AppFabricUser (machineName\username) | Domain Account, ex: oshyn.com\AppFabricDomainUser (domain\accountname) |
Lead Hosts\Cluster Management | Designated cache hosts (isLeadHost=true) serve as lead hosts that manage the cluster | SQL Server manages the Cluster |
Suitable Environments | Generally suitable for Development environments | Generally suitable for QA and Production Environments |
High Availability | As long as majority of lead hosts are up, the cluster is up. So if you have 4 nodes in the cluster, more than 2 nodes down will bring down the cluster. It also depends on usage of regions and some more conditions apply. | Since the SQL server manages cluster, as long as there is one cache server up, the cluster is up and running (it also depends on how the cluster is configured, high availability option, and presence of regions) |
Below is a pic that shows the options, this was for a cluster already created using XML Storage provider.
Once you click next, the next screen would provide you options to change the ports for the cache service. However, general leave the default values. As you can see from the above configuration wizard, you chose "New Cluster" when you are creating a new cache cluster with the cache server being part of that cluster. When you are adding additonal cache servers to the existing cluster, you just chose to "Join Cluster" and it will be part of the existing cluster.
You can enable the checkboxes for configuring firewall rules for AppFabric.
Common Commands for Windows Server AppFabric Distributed Caching Solution
In this section, we will see the various common commands that can be used with Windows AppFabric Distributed Caching Solution.
Let's talk about the common commands that help in this Distribution Cache Solution Land. All the commands for AppFabric are run in the windows powershell command tool. This can be launched by going to Start -> All Programs -> Windows Server App Fabric -> Caching Administration Windows PowerShell
To get all the commands related to cache
Get-Command *cache*
To get help on a command
Get-Help New-Cache -full
To create cache with Name "DEV_Cache"
New-Cache -CacheName DEV_Cache -TimeToLive 60 -Expirable true
To get cache
Get-Cache
To get cache host which provides the cache hosts and their status and the cacheserver host name and port on which it is running.
Get-CacheHost
To grant access to cache cluster (local and the domain scenarios)
Grant-CacheAllowedClientAccount -Account "NT AUTHORITY\NETWORK SERVICE"
Grant-CacheAllowedClientAccount -Account "domainname\machinename$"
To get client accounts that can access Cache
Get-CacheAllowedClientAccount
To Set cache-cluster security on the server, there are different levels are ProtectionLevel and SecurityMode.
Set-CacheClusterSecurity -ProtectionLevel None -SecurityMode None
To Start cache-cluster
Start-CacheCluster
To Stop cache-cluster
Stop-CacheCluster
To get cache Statistics, which provides details of no of misses, no of hits, no of items in cache, size of the cache and other details. Enter the following command and enter the cachename when prompted for it.
Get-CacheStatistics
To get cache cluster health, this provides the different parameters about state of the cluster like throttle and so on.
Get-CacheClusterHealth
There are several other commands that one can run for the cache cluster. Apart from this, there is a cache administration tool that one can use which allows to access cache through GUI.
Configuring Sitecore with Windows Server AppFabric Distributed Caching Solution and Cache Eviction using Sitecore Events
Now, let's get into Configuring Sitecore with Windows Server AppFabric Distributed Caching Solution, and how we can model the cache eviction based on CMS publishes by hooking into the Sitecore publish events. In this, we would discuss first integrating the cache cluster that is installed and configured, and then show how we can create publish handler which hooks into the publish events of sitecore and allows us to evict cache on publish of items.
Now let's get into the usage of the configured cache cluster with Sitecore. Configuration of Sitecore to use AppFabric Solution follows similar steps as configuration of .NET App to use AppFabric. Below is similar object code to help with this integration.
Caching Strategy
public class AppFabricCachePlatformManager {
private DataCacheFactory _DataCacheFactory = null;
public DataCacheFactory CacheFactory {
get
{
return _DataCacheFactory;
}
set
{
_DataCacheFactory = value;
}
}
private string _CacheName;
public string CacheName
{
get
{
return _CacheName;
}
set
{
_CacheName = value;
}
}
public AppFabricCachePlatformManager(string CachingHosts, string CacheName)
{
this.CacheName = CacheName;
List<DataCacheServerEndpoint> cacheCluster = new List<DataCacheServerEndpoint>();
//Assuming you have list of cache servers delimited by “;”
string[] cacheServers = CachingHosts.Split((new string[] { “;” }), StringSplitOptions.RemoveEmptyEntries);
if (cacheServers != null)
{
foreach (string cacheServer in cacheServers)
{
try
{
int serverNameLength = cacheServer.IndexOf(“:”);
string cacheServerName = cacheServer.Substring(0, serverNameLength);
int cacheServerPort = Convert.ToInt32(cacheServer.Substring(serverNameLength + 1));
cacheCluster.Add(new DataCacheServerEndpoint(cacheServerName, cacheServerPort));
}catch (Exception ex) {
throw new Exception(“Configuration key CacheHosts value entry should be of the format server1:port1;server2:port2;server3:port3;”, ex);
}
}
}
DataCacheFactoryConfiguration config = new DataCacheFactoryConfiguration();
config.Servers = cacheCluster;
config.LocalCacheProperties = new DataCacheLocalCacheProperties();
DataCacheSecurity security = new DataCacheSecurity(DataCacheSecurityMode.None, DataCacheProtectionLevel.None);
config.SecurityProperties = security;
}
public DataCache GetCache(string p_CacheName)
{
DataCache cache = null;
cache = this.CacheFactory.GetCache(p_CacheName);
return cache;
}
public object Get(string p_CacheKey, string p_CacheName)
{
this.GetCache(p_CacheName).Get(p_CacheKey);
}
public bool Remove(string p_CacheKey, string p_CacheName)
{
return this.GetCache(p_CacheName).Remove(p_CacheKey);
}
public bool Put(string p_CacheKey, object p_CacheValue)
{
return this.GetCache(p_CacheName).Put(p_CacheKey, p_CacheValue);
}
}
Now you can create an instance of this object and be able to integrate the cache cluster on your .NET app.
AppFabricCachePlatformManager appfabric = new AppFabricCachePlatformManager("OSHYN-PN:22233;OSHYN-PN2:22233", "DEV_Cache")
and this would connect to the DEV_Cache on the cache cluster. Now an object "product1" can be stored in cache as follows using the Put statement:
appfabric.Put("ProductKey_1", product1);
and this can be easily retrieved again as:
appfabric.Get("ProductKey_1");
Now assume this Product is an item being managed in Sitecore CMS. As such if the item content updates, then we need to flush this item from cache and and put it in cache the updated object. This is handled by hooking up into the Sitecore publish event and making sure this item is published.
Eviction Strategy
The eviction strategy consits of clearing the item from cache when the item gets updated on sitecore and is published. We can add an event handler for publish event and clear cache on publish. Creation of the Publish Handler is as follows:
namespace Company.Domain.Sitecore.Publishing
{
public class PublishHandler
{
public void OnItemPublished(object sender, EventArgs args)
{
global::Sitecore.Diagnostics.Log.Debug(String.Format(“Started Publish handler OnItemPublished”));
if (args == null)
{
global::Sitecore.Diagnostics.Log.Debug(String.Format(“Finished Publish handler OnItemPublished args=null”));
return;
}
global::Sitecore.Publishing.Pipelines.PublishItem.ItemProcessedEventArgs theArgs = args as global::Sitecore.Publishing.Pipelines.PublishItem.ItemProcessedEventArgs;
global::Sitecore.Data.Items.Item currentItem = theArgs.Context.PublishHelper.GetSourceItem(theArgs.Context.ItemId);
if ((currentItem != null) && (currentItem.Paths != null) && (currentItem.Paths.IsContentItem))
{
//Get the product template
string productTemplateGUID = “{AB9791FE-EF31-4BC5-D9EF-8B886C928ACB}”;
global::Sitecore.Data.ID productTemplateID = new global::Sitecore.Data.ID(productTemplateGUID);
global::Sitecore.Diagnostics.Log.Debug(String.Format(“productTemplateID is not null”));
if (!currentItem.TemplateID.IsNull && !productTemplateID.IsNull && currentItem.TemplateID == productTemplateID)
{
global::Sitecore.Diagnostics.Log.Debug(String.Format(“Product Item with ID={0} has been published”, currentItem.ID.ToString()));
//assuming the key is build using sitecore itemid whne storing in cache layer
//if there is some external key that you are using to store in cache,
//then you need to have a Dictionary mapping of sitecore item ids to that
//external key and use that instead of currentItem.ID.ToString();
CacheManager.Instance.CachePlatform.Remove(“ProductKey_” + currentItem.ID.ToString());
global::Sitecore.Diagnostics.Log.Debug(String.Format(“Product Item with ID={0} has been removed from titles cache”, currentItem.ID.ToString()));
}
}
global::Sitecore.Diagnostics.Log.Debug(String.Format(“Finished Publish handler OnItemPublished”));
}
}
}
Modifying the web.config to take trigger this handler on publish event. In the "<events>" section of the web.config of sitecore, add this:
<event name=“publish:itemProcessed” help=“Receives an argument of type ItemProcessedEventArgs (namespace: Sitecore.Publishing.Pipelines.PublishItem)” >
<handler type=“Company.Domain.Sitecore.Publishing.PublishHandler, CompanyDLL” method=“OnItemPublished” />
</event>
This would trigger the publish handler once publish engine finishes processing the item on publish.
In this way, you can check if product item that is cached is modified and can evict it from cache when the item gets updated and published on the sitecore cms side. This event hook up methodology can be used in other forms of events exposed by sitecore.