
Websites on Smartphones & Tablets: Using Touch Events for Image Scrolling
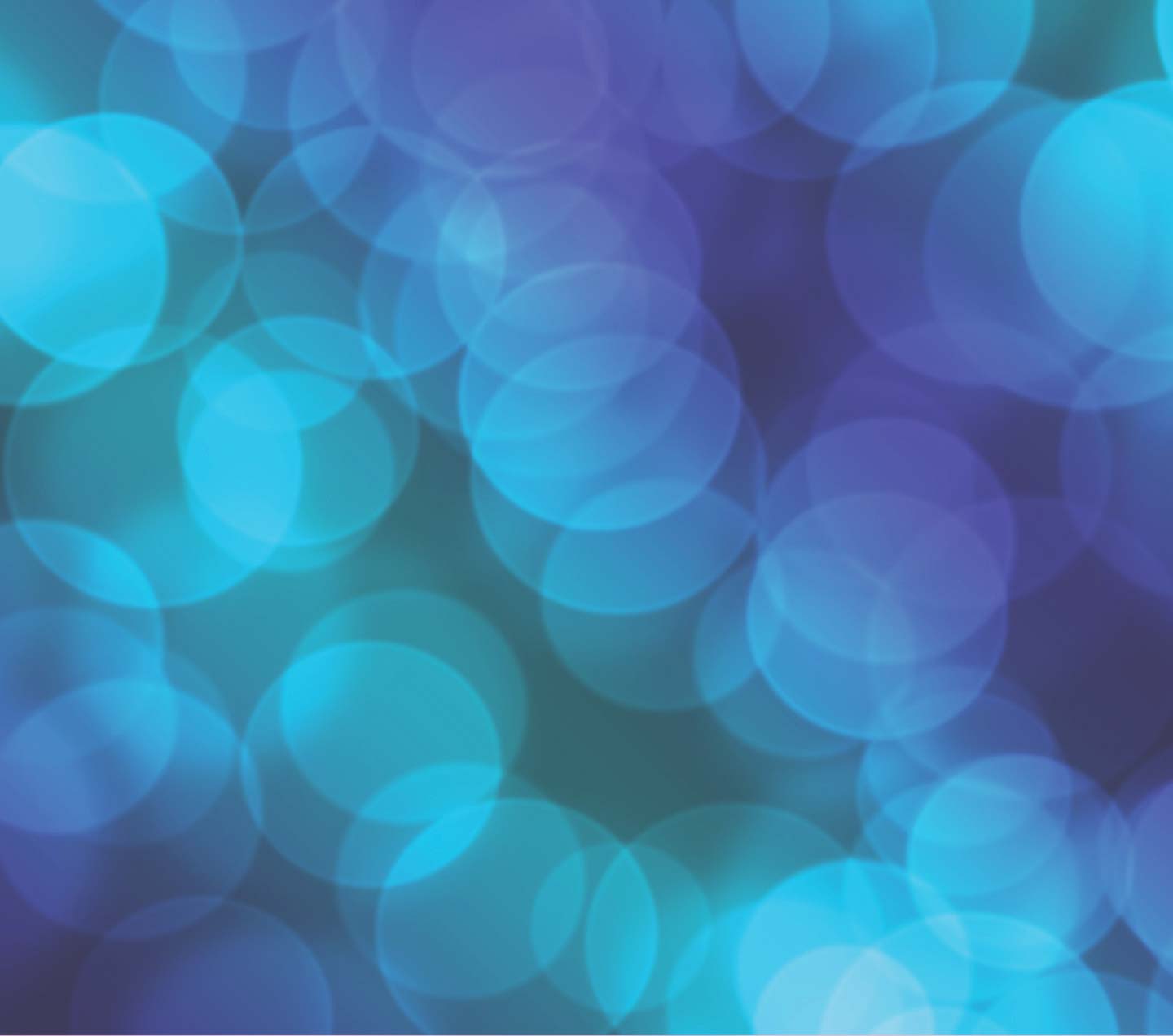


Nov 21, 2011
With the amount of mobile browsing growing daily, it is important to make sure that your website is compatible on all browsers (or at least with the majority). In the weekend after the new iPhone 4S release, Apple was able to sell 4 million devices1. With numbers like this, it’s important to take the iPhone and iPad into account when creating your website.
Your website must be fully compatible with these devices and the user should be able to navigate around the website without any trouble.
Using jQuery plugins, we can add custom functions to ‘touch events’ to make a simple image scroller.
Touch Events
In the iPhone and iPad, the mouse events are replaced by touch events. These events are:
touchstart: it is triggered when a touch is initiated. Mouse equivalent - mouseDown
touchmove: it is triggered when a touch moves. Mouse equivalent - mouseMove
touchend: it is triggered when a touch ends. Mouse equivalent - mouseUp.
touchcancel: it is triggered when the system cancels tracking for the touch.
When you overwrite some of these events, the event listener will receive an object of type event. This object has some important properties:
- touches: is an object collection of type touch. These objects have the properties pageY and pageX that contain the coordinates of the object within the page.
- targetTouches: works as touches, but it concerns only to one item instead of the entire page.
Recently a W3C working group came together to work on this touch events specification guideline.
Image Scroller
To implement a simple image scroller we are going to use two jQuery plugins: TouchSwipe and Cycle
TouchSwipe is a jQuery plugin used on touch input devices such as the iPad and iPhone. It enables the detection of swipe gestures.
Download the TouchSwipe plugin.
Cycle is a slideshow plugin that supports many different types of transition effects.
Download the Cycle plugin.
First, we would need to include the jQuery library as well as TouchSwipe and Cycle plugins:
<!DOCTYPE html>
<html lang="es">
<head>
<script src="js/jquery.min.js" type="text/javascript"></script>
<script type="text/javascript" src="js/jquery.touchSwipe-1.2.5.js"></script>
<script type="text/javascript" src="js/jquery.cycle.all.min.js"></script>
</head>
<body>
</body>
</html>
After that we will need to add the HTML structure:
<!DOCTYPE html>
<html lang="es">
<head>
<script src="js/jquery.min.js" type="text/javascript"></script>
<script type="text/javascript" src="js/jquery.touchSwipe-1.2.5.js"></script>
<script type="text/javascript" src="js/jquery.cycle.all.min.js"></script>
</head>
<body>
<div>
<div>
<h1>swipe the image</h1>
</div>
<div class="content" id="demos">
<div id="slideshow" class="pics">
<img alt="" src="../../Images/logo001.png" />
<img alt="" src="../../Images/logo002.png" />
<img alt="" src="../../Images/logo003.png" />
</div>
</div>
</div>
</body></html>
In the body structure we only have an h1 title and a container that holds the images that are going to be part of the slide show.
Now we have to define the JavaScript code:
<!DOCTYPE html>
<html lang="es">
<head>
<script src="js/jquery.min.js" type="text/javascript"></script>
<script type="text/javascript" src="js/jquery.touchSwipe-1.2.5.js"></script>
<script type="text/javascript" src="js/jquery.cycle.all.min.js"></script>
<script type="text/javascript">
$(function() {
$('#slideshow').cycle({
fx: 'scrollHorz',
timeout: 0
});
//set up swipe events for main set
$('#slideshow').swipe({
swipeLeft: function(){
$('#slideshow').cycle('next');
},
swipeRight: function(){
$('#slideshow').cycle('prev');
}
});
});
</script>
</head>
<body>
<div>
<div>
<h1>swipe left/right the image</h1>
</div>
<div class="content" id="demos">
<div id="slideshow" class="pics">
<img alt="" src="http://oshyn.com/Images/logo001.png" />
<img alt="" src="http://oshyn.com/Images/logo002.png" />
<img alt="" src="http://oshyn.com/Images/logo003.png" />
</div>
</div>
</div>
</body>
</html>
The code will:
- Set up the instance of the Cycle plugin:
$('#slideshow').cycle({
fx: 'scrollHorz',
timeout: 0
});
This code also set up the effect used as a transition when the image is changed
Set up the instance of the TouchSwipe plugin:
$('#slideshow').swipe({
swipeLeft: function(){
$('#slideshow').cycle('next');
},
swipeRight: function(){
$('#slideshow').cycle('prev');
}
});
In this code also we define the function that has to be executed when a swipe left or right is triggered. For a left swipe we will define it to go next image and for a right swipe, we will define it to go the previous image.
If we want to implement up/down swipes, we will have to change to this code:
$('#slideshow').cycle({
fx:'scrollVert',
timeout: 0
});
$('#slideshow').swipe({
swipeDown: function(){
$('#slideshow').cycle('next');
},
swipeUp: function(){
$('#slideshow').cycle('prev');
}
});
In conclusion, TouchSwipe creates a simple way to capture swipes events in mobile devices and, combined with the Cycle plugin, can be very useful to develop mobile web pages.