
Importing Documents into OpenText Content Server
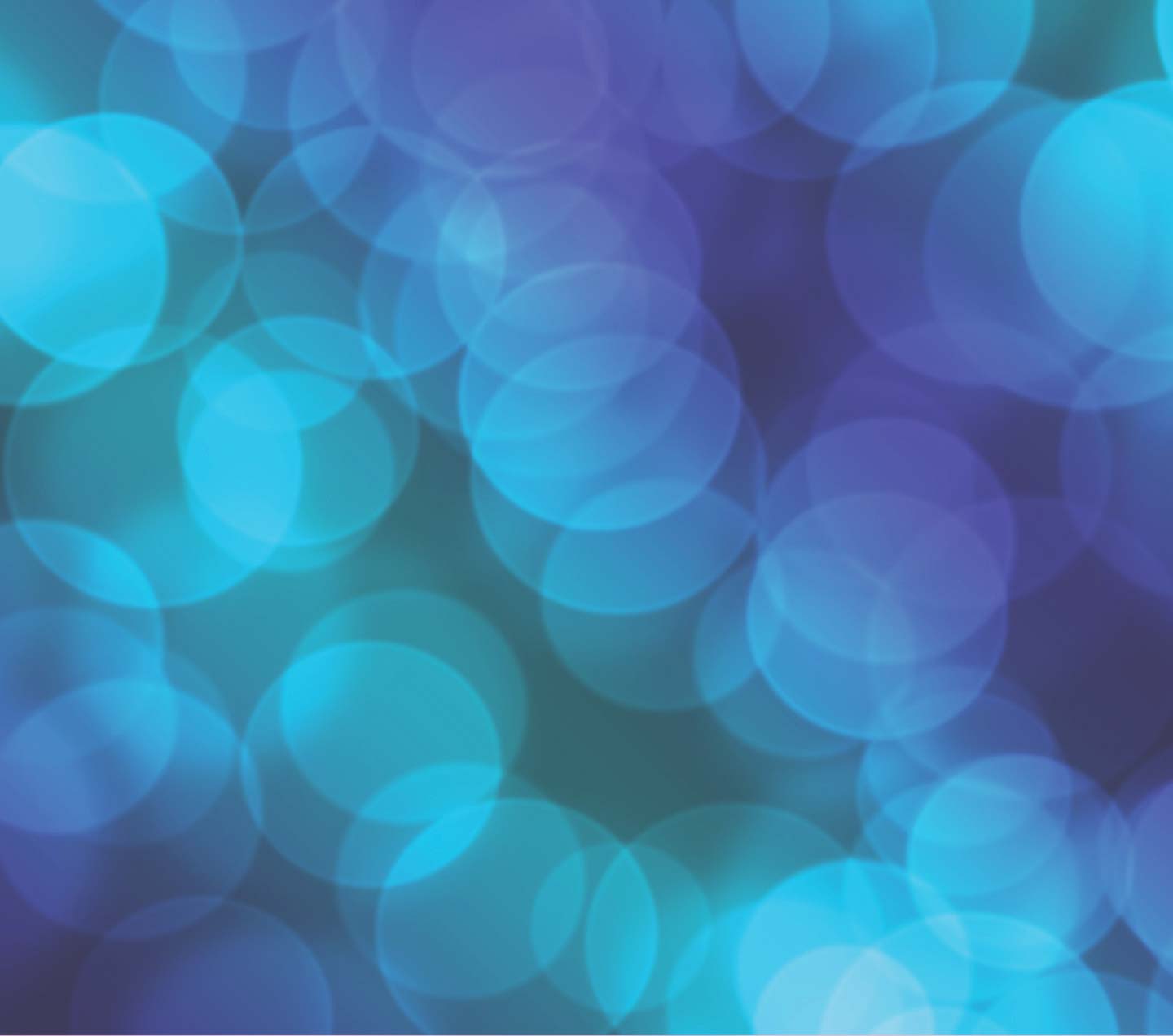


Nov 09, 2011
Adding or importing documents into Content Server is a very simple task, but it could turn into a painful one when you have hundreds or even thousands of documents to import. OpenText has provided a nice tool to help us automate this job; it’s called the Object Importer module.
Using the OpenText Object Importer
Object Importer enables the automatic import of any number of objects from the local file system into Content Server. The control file that manages the process is configurable. Within the control file, you specify where in Content Server to upload the objects and where the objects to be uploaded are located on the file system. You also have full control over each object's permissions, title, attributes, and other object metadata within Content Server.
First, you will need to download and install this module. You can download Object Importer v.10.0.0 here. Please follow the instructions provided to install and configure the Object Importer module.
Object Importer can create, update, or delete objects within Content Server based on directions from an import control file. The import control file contains meta information supplied by the user. It is an XML file, and must contain valid XML. The import control file can have any name and ASCII extension, for example, import.txt.
It is very important that the control file be properly formatted. The import will fail unless the entire import control file, including comments, is contained within the import tags, <import></import>.
Between the <import></import> tags are multiple paragraphs, called node paragraphs. Each node paragraph describes one action against a particular object. Each node paragraph begins with a <node> tag and ends with a </node> tag.
In order to automatically import documents from a specific folder, you will need to create a control file containing one node per document you wish to import. Each node must have at least the location where you want to put the file in Content Server and the path where the file is located in the file system. For example, you can have a control file with the following structure:
<import>
<node type="document" action="create">
<title>Document1</title>
<location>Enterprise:Import Folder</location>
<file>C:\Documents\File1.pdf</file>
</node>
<node type="document" action="create">
<title>Document2</title>
<location>Enterprise:Import Folder</location>
<file>C:\Documents\File2.pdf</file>
</node>
...
<node type="document" action="create">
<title>DocumentN</title>
<location>Enterprise:Import Folder</location>
<file>C:\Documents\FileN.pdf</file>
</node>
</import>
This control file will import all the documents to a folder called Import Folder under the Enterprise Workspace. In case this folder does not exist, Object Importer will create it for you.
Below is a simple method written in C# that you can use to generate a control file containing all the files in a specified directory passed as a constant argument.
void GenerateControlFile()
{
var stringBuilder = new StringBuilder();
var xmlSettings = new XmlWriterSettings {
Encoding = Encoding.UTF8,
OmitXmlDeclaration = true,
Indent = true };
//Path to the folder where the files reside
// Ex. "C:\Documents"
const string currentFolder = @"";
//Content Server location where files will be imported.
// Ex. "Enterprise:Folder01:InnerFolder"
const string csLocation = "";
var directoryInfo = new DirectoryInfo(currentFolder);
using (XmlWriter xmlWriter = XmlWriter.Create(
stringBuilder,
xmlSettings))
{
xmlWriter.WriteStartElement("import");
foreach (FileInfo file in directoryInfo.GetFiles())
{
string title = Path.GetFileNameWithoutExtension(file.Name);
xmlWriter.WriteStartElement("node");
xmlWriter.WriteAttributeString("type", "document");
xmlWriter.WriteAttributeString("action", "create");
xmlWriter.WriteStartElement("title");
xmlWriter.WriteAttributeString("language", "en_US");
xmlWriter.WriteString(title);
xmlWriter.WriteEndElement();
xmlWriter.WriteElementString("location", csLocation);
xmlWriter.WriteElementString("file", file.FullName);
xmlWriter.WriteEndElement(); //node
}
xmlWriter.WriteEndElement(); //import
xmlWriter.Flush();
using (StreamWriter sw = File.CreateText(@"C:\control-file.xml"))
{
sw.WriteLine(stringBuilder.ToString());
}
Console.WriteLine("\nFile successfully created.\n");
}
}
Once you have the Control File created, simply go to the Content Server Administration Interface and choose Manual Object Import under the Object Importer Administration menu. On that screen you need to specify a Name for the log file (it cannot contain any spaces) and browse for the Control File you have already created.
Object Importer will import all the documents contained in the Control File to the folder specified under the location tags.