
Easy way to provide in-application iPhone Emailing
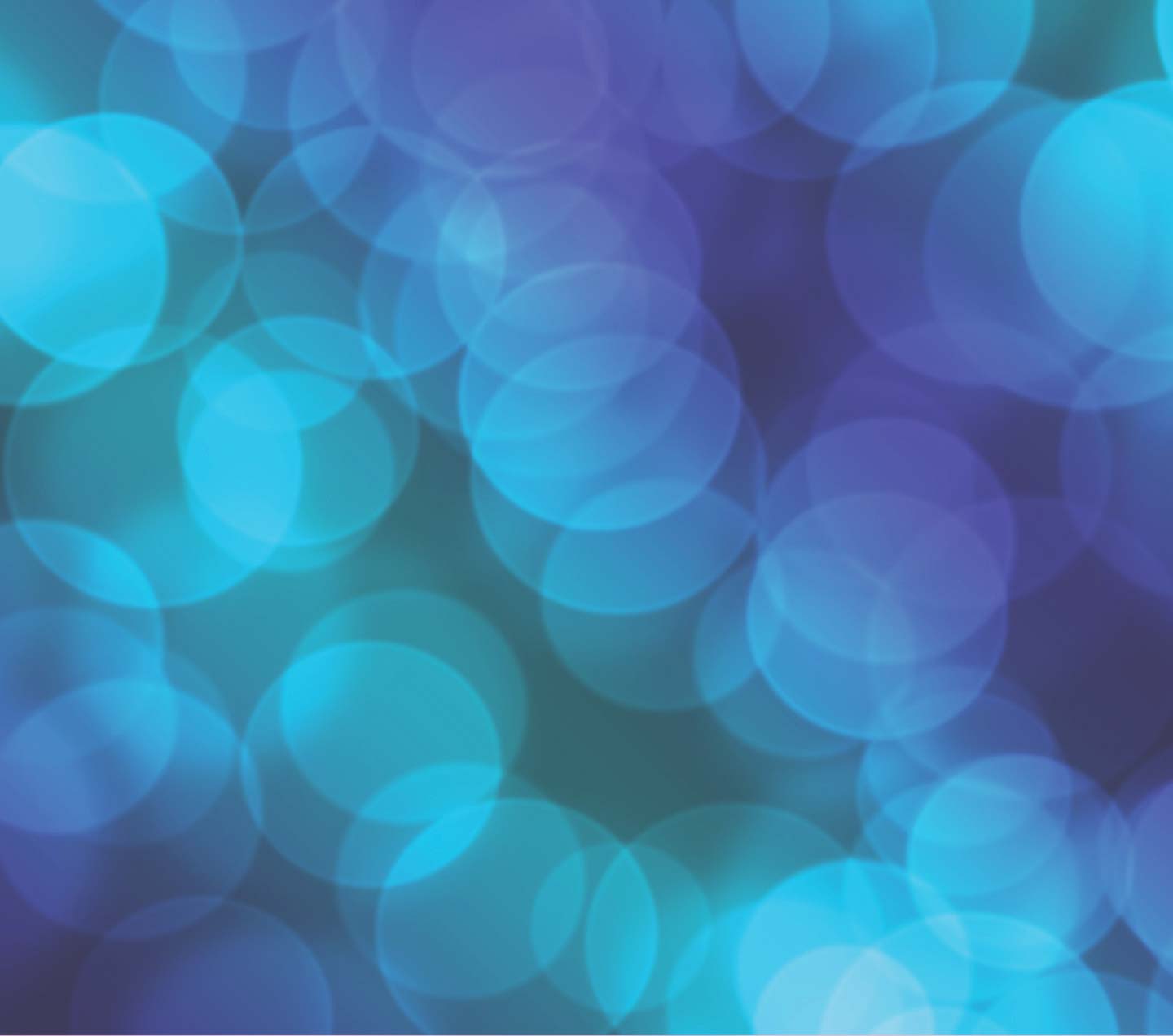


Nov 26, 2009
Most of all applications available in the Apple App Store provides us the "Send Email", "Recommend a Friend" and "Share This" functionality. There are two different ways to provide you applications this feature: the hard one (which is usually the wrong one too, believe me I've seen coding horrors in this subject) and the simple one (the correct one).
First, to program iPhone applications you MUST know every framework provided by the iPhone SDK like you know yourself. If you not, you are prone to make awful design mistakes which leads to performance and maintainability issues. For this post purpose we will use the MessageUI framework.
-Starting Point
Open Xcode and create new project. You must choose the "New View Based Application" option.
Once the new project is created, we must now import the MesageUI Framework. To do that simply go to your "Frameworks" folder, right click one of the frameworks and choose the "Reveal in Finder" option. This will allow you to browse all frameworks in the Finder. Locate the "MessageUI.framework" file. Once located, drag it to your "Frameworks" folder in the Xcode project. Don't forget to right click the MessageUI.framework and click the Add option and make sure the "Copy items into destination group's folder (if needed)" is NOT checked. This tip also applies for any other framework you need to import for your app.
-Writing the Code-
Now that our base project is ready it's time to go big time. Go to the MyViewController.h file and create an IBAction. This is the action that will be binded to a Button control in our View. Repeat the same step in the MyViewController.m file. You can give your files whatever name you want as long as the .h and .m implementation files are the same. Now go to the Interface Builder and add a Button control. We must declare an import directive in our .h file that references the MessageUI Framework. To do that, write the following line to you .h file:
#import <MessageUI/MessageUI.h>
Now we need to also put in the delegate protocols for this framework. This means build the base interface which we will implement in the .m file. The final MyViewController.h file must look like this (with the IBAction for the button):
#import
@interface MailComposerViewController : UIViewController
{
}
-(IBAction)pushEmail;
@end
Now that you have the header file we have to implement it in the MyViewController.m file. Here we will implement our sendMail method. Put this code into the MyViewController.m file:
-(IBAction)pushEmail {
MFMailComposeViewController *mail = [[MFMailComposeViewController alloc] init];
mail.mailComposeDelegate = self;
if ([MFMailComposeViewController canSendMail]) {
//Setting up the Subject, recipients, and message body.
[mail setToRecipients:[NSArray arrayWithObjects:@"email@email.com",nil]];
[mail setSubject:@"Subject of Email"];
[mail setMessageBody:@"Message of email" isHTML:NO];
//Present the mail view controller
[self presentModalViewController:mail animated:YES];
}
//release the mail
[mailComposer release];
}
//This is one of the delegate methods that handles success or failure
//and dismisses the mail
- (void)mailComposeController:(MFMailComposeViewController*)controller didFinishWithResult:(MFMailComposeResult)result error:(NSError*)error
{
[self dismissModalViewControllerAnimated:YES];
if (result == MFMailComposeResultFailed) {
UIAlertView *alert = [[UIAlertView alloc] initWithTitle:@"Message Failed!"" message:@"Your email has failed to send" delegate:self cancelButtonTitle:@"Dismiss" otherButtonTitles:nil];
[alert show];
[alert release];
}
}
Voilà, build and test the project and you have a ready to use emailing controller you can use inside you applications.
-Extra Credit-
What if we need attachments? "Send this photo to a friend" maybe? No problems. It's really easy and this is how: Under the code line where you add the email recipient use this piece of code:
UIImage *picture = [UIImage imageNamed:@"MyPic.png"];
NSData *data = UIImageJPEGRepresentation(pic ,1.0);
[mail addAttachmentData:data mimeType:@"image/jpeg" fileName:@"MyPic.jpeg"];
We set up the MailComposer control in the first part of the code in the action implementation. Then we call a didFinishWithResult method where we are setting up if the email fails or sends. Also, it sets up a Cancel button for you too. In the attachment section just edit the imageNamed:@" with your images names/paths.
Recent Insights
-
-
-
Fernando Torres
Tailwind CSS in Modern Web Development
Integration with AEM, Sitecore, and Optimizely
-